Amazonで入手した1.8インチLCD
[WINGONEER 1.8インチフルカラー128×160 SPIフルカラーTFT LCDディスプレイモジュールST7735S 3.3V DIYキット用OLED電源の交換]
https://amzn.asia/d/ecQo4Wy
をRaspberry Pi Picoで動かすテストコード
1フレーム描画に50msecぐらいかかる。
ST7735SはSPI33MHzまで使えるようなのでもう少し早くはできそう
通信にすきまもあるしがんばれば30FPSいけそう
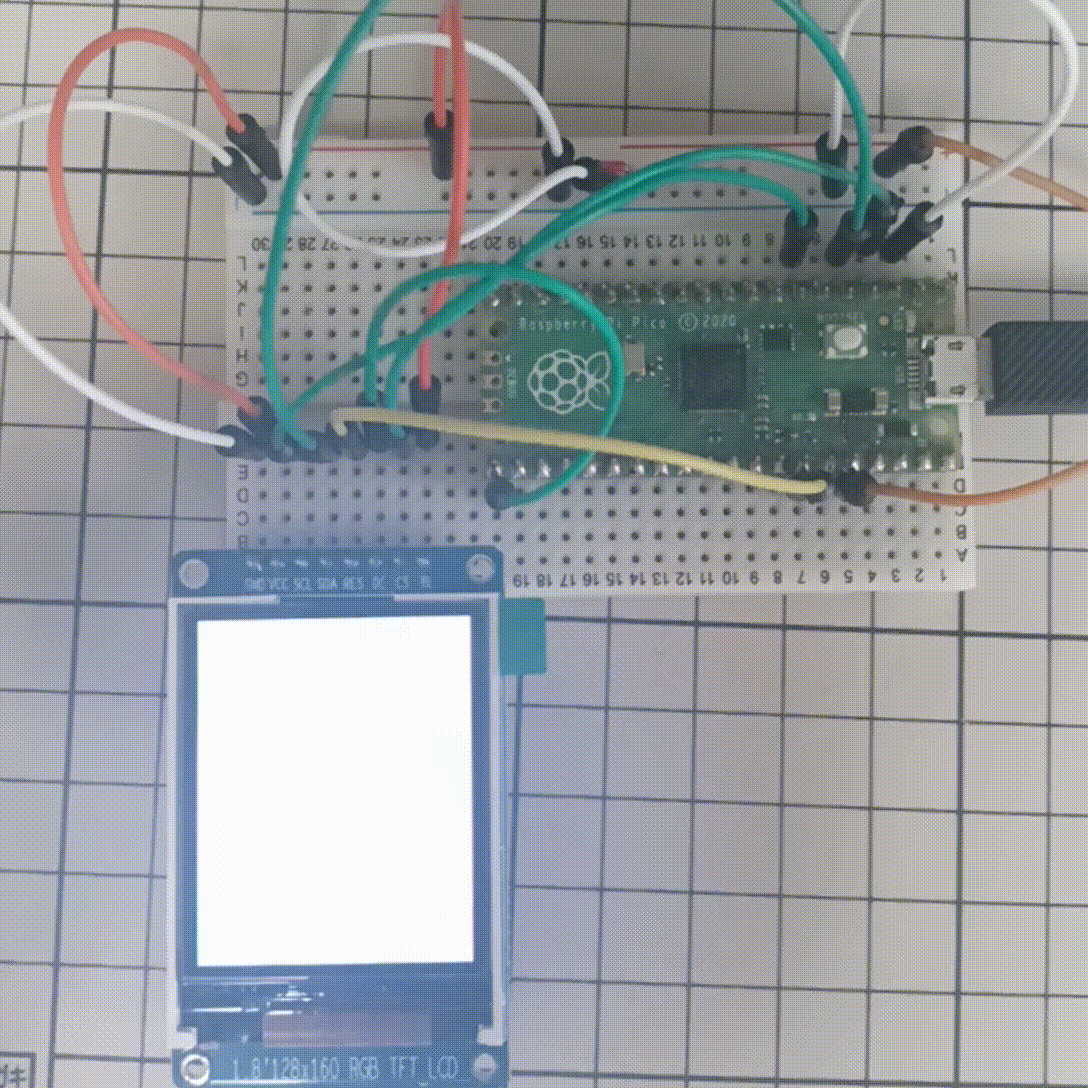
#include <stdio.h>
#include "pico/stdlib.h"
#include "hardware/spi.h"
// SPI Defines
// We are going to use SPI 0, and allocate it to the following GPIO pins
// Pins can be changed, see the GPIO function select table in the datasheet for information on GPIO assignments
#define LCD2_SPI_CH ( spi0 )
#define P_LCD2_SCK ( 2 )
#define P_LCD2_TX ( 3 )
#define P_LCD2_RX ( 4 ) //未使用
#define P_LCD2_CS ( 5 )
#define P_LCD2_CD ( 16 )
#define P_LCD2_RESET ( 28 )
volatile static uint16_t lcd2_timer_1ms = 0;
void Lcd2TimerInc( void )
{
if ( lcd2_timer_1ms < 0xFFFF )
{
lcd2_timer_1ms++;
}
}
// 1msec割り込み
static bool __isr __time_critical_func(timer_callback)( repeating_timer_t *rt )
{
Lcd2TimerInc();
return ( true );
}
#define LCD2_SEND_CMD (0)
#define LCD2_SEND_DATA (1)
static void Lcd2Send( uint8_t is_data, uint8_t data )
{
gpio_put( P_LCD2_CD , is_data );
{
uint8_t buf[1];
buf[0]=data;
spi_write_blocking( LCD2_SPI_CH, buf, 1 );
}
}
void display(unsigned char d, unsigned char d1)
{
uint16_t i, j;
for( j = 0u ; j < 162u; j++ )
{
for( i = 0u; i < 132u; i++ )
{
Lcd2Send( LCD2_SEND_DATA, d );
Lcd2Send( LCD2_SEND_DATA, d1 );
}
}
}
int main()
{
stdio_init_all();
// SPI initialisation. This example will use SPI at 1MHz.
spi_init( LCD2_SPI_CH, 25 * 1000 * 1000 );
spi_set_format( LCD2_SPI_CH, 8, SPI_CPOL_0, SPI_CPHA_0, SPI_MSB_FIRST );
gpio_set_function( P_LCD2_RX, GPIO_FUNC_SPI );
gpio_set_function( P_LCD2_SCK, GPIO_FUNC_SPI );
gpio_set_function( P_LCD2_TX, GPIO_FUNC_SPI );
gpio_init( P_LCD2_CS );
gpio_init( P_LCD2_CD );
gpio_init( P_LCD2_RESET );
// Chip select is active-low, so we'll initialise it to a driven-high state
gpio_set_dir( P_LCD2_CS , GPIO_OUT );
gpio_set_dir( P_LCD2_CD , GPIO_OUT );
gpio_set_dir( P_LCD2_RESET, GPIO_OUT );
gpio_put( P_LCD2_CS, 1 );
// タイマ初期設定
{
static repeating_timer_t timer;
/* 1msインターバルタイマ設定 */
add_repeating_timer_us( -1000, &timer_callback, NULL, &timer );
}
// ハードウェアRESET
{
gpio_put( P_LCD2_RESET , 1 );
lcd2_timer_1ms = 0;
while ( lcd2_timer_1ms < 100 ){}
gpio_put( P_LCD2_RESET , 0 );
lcd2_timer_1ms = 0;
while ( lcd2_timer_1ms < 100 ){}
gpio_put( P_LCD2_RESET , 1 );
lcd2_timer_1ms = 0;
while ( lcd2_timer_1ms < 100 ){}
}
/* ポート初期化 */
{
gpio_put( P_LCD2_CS , 1 );
gpio_put( P_LCD2_CS , 0 );
}
// 初期化
{
Lcd2Send( LCD2_SEND_CMD, 0x11 );
lcd2_timer_1ms = 0;
while ( lcd2_timer_1ms < 200 ){}
Lcd2Send( LCD2_SEND_CMD, 0xEC );
Lcd2Send( LCD2_SEND_DATA, 0x02 ); //0x0C
Lcd2Send( LCD2_SEND_CMD, 0x26 ); //Set Default Gamma
Lcd2Send( LCD2_SEND_DATA, 0x04 );
Lcd2Send( LCD2_SEND_CMD, 0xB1 );
Lcd2Send( LCD2_SEND_DATA, 0x08 );
Lcd2Send( LCD2_SEND_DATA, 0x14 );
Lcd2Send( LCD2_SEND_CMD, 0xC0 ); //Set VRH1[4:0] & VC[2:0] for VCI1 & GVDD
Lcd2Send( LCD2_SEND_DATA, 0x08 );
Lcd2Send( LCD2_SEND_DATA, 0x00 );
Lcd2Send( LCD2_SEND_CMD, 0xC1 ); //Set BT[2:0] for AVDD & VCL & VGH & VGL
Lcd2Send( LCD2_SEND_DATA, 0x05 );
Lcd2Send( LCD2_SEND_CMD, 0xC5 ); //Set VMH[6:0] & VML[6:0] for VOMH & VCOML
Lcd2Send( LCD2_SEND_DATA, 0x46 );//0x46
Lcd2Send( LCD2_SEND_DATA, 0x40 );//0x40
Lcd2Send( LCD2_SEND_CMD, 0xC7 );
Lcd2Send( LCD2_SEND_DATA, 0xBD ); //0xC2
Lcd2Send( LCD2_SEND_CMD, 0x3a ); //Set Color Format
Lcd2Send( LCD2_SEND_DATA, 0x05 );
Lcd2Send( LCD2_SEND_CMD, 0x2A ); //Set Column Address
Lcd2Send( LCD2_SEND_DATA, 0x00 );
Lcd2Send( LCD2_SEND_DATA, 0x00 );
Lcd2Send( LCD2_SEND_DATA, 0x00 );
Lcd2Send( LCD2_SEND_DATA, 0x83 );
Lcd2Send( LCD2_SEND_CMD, 0x2B ); //Set Page Address
Lcd2Send( LCD2_SEND_DATA, 0x00 );
Lcd2Send( LCD2_SEND_DATA, 0x00 );
Lcd2Send( LCD2_SEND_DATA, 0x00 );
Lcd2Send( LCD2_SEND_DATA, 0xA1 );
Lcd2Send( LCD2_SEND_CMD, 0xB4 ); //Set Source Output Direction
Lcd2Send( LCD2_SEND_DATA, 0x06 );
Lcd2Send( LCD2_SEND_CMD, 0xf2 ); //Enable Gamma bit
Lcd2Send( LCD2_SEND_DATA, 0x01 );
Lcd2Send( LCD2_SEND_CMD, 0x36 ); //Set Scanning Direction
Lcd2Send( LCD2_SEND_DATA, 0xC0 ); //0xc0
Lcd2Send( LCD2_SEND_CMD, 0xE0 );
Lcd2Send( LCD2_SEND_DATA, 0x3F );//p1
Lcd2Send( LCD2_SEND_DATA, 0x26 );//p2
Lcd2Send( LCD2_SEND_DATA, 0x23 );//p3
Lcd2Send( LCD2_SEND_DATA, 0x30 );//p4
Lcd2Send( LCD2_SEND_DATA, 0x28 );//p5
Lcd2Send( LCD2_SEND_DATA, 0x10 );//p6
Lcd2Send( LCD2_SEND_DATA, 0x55 );//p7
Lcd2Send( LCD2_SEND_DATA, 0xB7 );//p8
Lcd2Send( LCD2_SEND_DATA, 0x40 );//p9
Lcd2Send( LCD2_SEND_DATA, 0x19 );//p10
Lcd2Send( LCD2_SEND_DATA, 0x10 );//p11
Lcd2Send( LCD2_SEND_DATA, 0x1E );//p12
Lcd2Send( LCD2_SEND_DATA, 0x02 );//p13
Lcd2Send( LCD2_SEND_DATA, 0x01 );//p14
Lcd2Send( LCD2_SEND_DATA, 0x00 );//p15
Lcd2Send( LCD2_SEND_CMD, 0xE1 );
Lcd2Send( LCD2_SEND_DATA, 0x00 );//p1
Lcd2Send( LCD2_SEND_DATA, 0x19 );//p2
Lcd2Send( LCD2_SEND_DATA, 0x1C );//p3
Lcd2Send( LCD2_SEND_DATA, 0x0F );//p4
Lcd2Send( LCD2_SEND_DATA, 0x14 );//p5
Lcd2Send( LCD2_SEND_DATA, 0x0F );//p6
Lcd2Send( LCD2_SEND_DATA, 0x2A );//p7
Lcd2Send( LCD2_SEND_DATA, 0x48 );//p8
Lcd2Send( LCD2_SEND_DATA, 0x3F );//p9
Lcd2Send( LCD2_SEND_DATA, 0x06 );//p10
Lcd2Send( LCD2_SEND_DATA, 0x1D );//p11
Lcd2Send( LCD2_SEND_DATA, 0x21 );//p12
Lcd2Send( LCD2_SEND_DATA, 0x3D );//p13
Lcd2Send( LCD2_SEND_DATA, 0x3E );//p14
Lcd2Send( LCD2_SEND_DATA, 0x3F );//p15
Lcd2Send( LCD2_SEND_CMD, 0x29 ); //Display on
Lcd2Send( LCD2_SEND_CMD, 0x2c ); // write data
}
// 描画
while ( 1 )
{
display( 0xf8, 0x00 ); //red color
lcd2_timer_1ms = 0; while ( lcd2_timer_1ms < 1000 ){}
display( 0x00, 0x00 ); //black color
lcd2_timer_1ms = 0; while ( lcd2_timer_1ms < 1000 ){}
display( 0xff, 0xff ); //white color
lcd2_timer_1ms = 0; while ( lcd2_timer_1ms < 1000 ){}
display( 0xf8, 0x00 ); //red color
lcd2_timer_1ms = 0; while ( lcd2_timer_1ms < 1000 ){}
display( 0x07, 0xe0 ); //green color
lcd2_timer_1ms = 0; while ( lcd2_timer_1ms < 1000 ){}
display( 0x00, 0x1f ); //blue color
lcd2_timer_1ms = 0; while ( lcd2_timer_1ms < 1000 ){}
display( 0x00, 0x00 ); //black color
lcd2_timer_1ms = 0; while ( lcd2_timer_1ms < 1000 ){}
}
return 0;
}
コメント